6.2.5 - SUI Buttons
In SUI we have two types of buttons:
- SButton: a text button like "Single player", "Multiplayer", "Options", "Exit" of the main menu
- SBgButton: a classic button with a colored background
A button executes whatever we say it to do when it's pressed.
Text buttons
To create one we can do like below and use .Notify to specify what needs to be done when pressed, by passing it a method which takes no parameters:
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Anchor(AnchorType.Fill)
- SButton.Text("Text Button").Notify(() => { RLog.Msg("text button pressed"); }); // adding a text button to the container which when pressed prints text in the console
panel.Add(container);
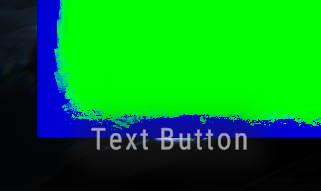
Background buttons
To create one we can do like below, always using .Notify to specify what to execute when pressed:
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Anchor(AnchorType.Fill)
- SBgButton.Text("Text Button").FontColor(Color.black).Notify(() => { RLog.Msg("text button pressed"); }); // adding a background button to the container with a black font color
panel.Add(container);
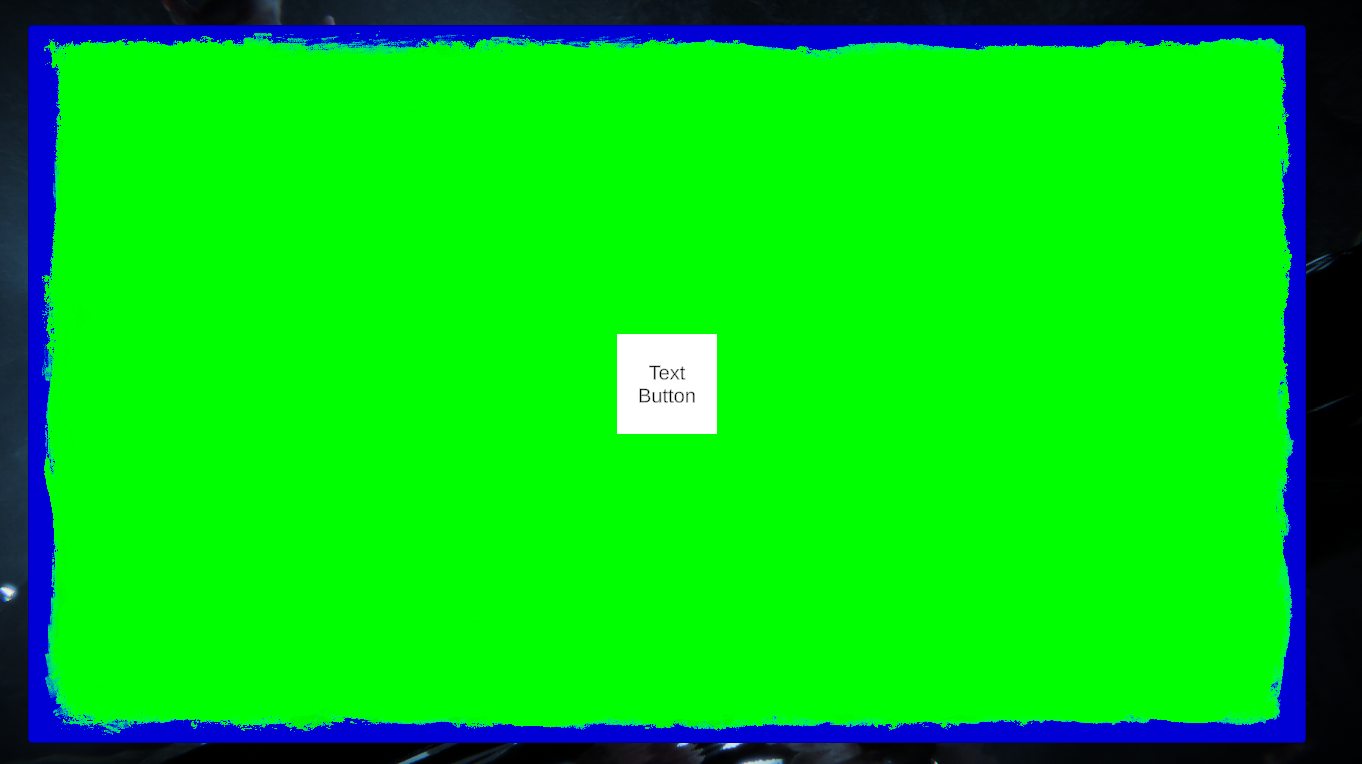
Passing a parameter to a button
To pass a parameter to the method that it's executed when the button is clicked we can do like so:
public static void Create()
{
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Anchor(AnchorType.Fill)
- SBgButton.Text("Text Button").Notify(() => PrintNumber(10)); // passing the 10 as the int parameter
panel.Add(container);
}
private static void PrintNumber(int number)
{
RLog.Msg(number);
}
Now everytime the button is clicked it will print 10 in the console.
Styling background buttons
The default color is white and the default background style is EBackground.None. We can change their color with .Color and their style using .Background passing the EBackground enum value just like when styling panels and containers.